Java Syntax Related Changes Since Java SE 6
August 22, 2014 ·
In this post I am going to discuss about JDK related changes since version 6 and how it may affect Android development.
What’s New in Java SE 7 Syntax
Binary Literals
We may display 0b or 0B in front of a number to indicate a binary number. E.g.
1int aNum = 0b00100001 // 33Underscore in Numeric Literals
We can separate a group of numbers with underscore in between digits to make it more readable, and the actual value is the same as without underscore
1long ccNumber = 1111_1111_1111_1111L // which is the same as 1111111111111111LNote
- We cannot add underscore before and after decimal point
- We cannot add underscore as a prefix to a number (this make it as identifier and not a numeric literal)
- We cannot add underscore as a suffix to a number
- We cannot add underscire in the 0x radix prefix
String in Switch Statement
In Java SE 6 if we want to do a conditional statement on a String we need to do it in a if…else Statement
1if(a.equals("apple")) {2 // do something3} else if (a.equals("orange")) {4 // do another thing5} else if (a.equals("pear")) {6 // do some other thing7} else {8 // do something when above conditions do not match9}In Java SE 7 we can wrap it into a Switch Statement
1switch(a) {2 case "apple":3 //do something4 break;5 case "orange":6 // do something7 break;8 case "pear":9 // do somethin10 break;11 default:12 // do something13 break;14}Type Inference for Generic instance Creation (a.k.a Diamond)
In Java SE 6 we can create a generic collection like a list of Strings in the following syntax
1List<String> strCollections = new ArrayList<String>();In Java SE 7 we can create the same list with a shorter syntax
1List<String> strCollections = new ArrayList<>();Improved Compiler Warnings and Errors When Using Non-Reifiable Formal Parameters with Varargs Methods
see http://docs.oracle.com/javase/7/docs/technotes/guides/language/non-reifiable-varargs.html for more details.
Try-with-Resources
Java SE 6
1BufferedReader br;2try {3 br = new BufferedReader(...);4 br.readLine();5} catch (IOException e) {6 // do something7} finally {8 if (br != null) {9 br.close();10 }11}Java SE 7
1try(BufferedReader br = new BufferedReader(...)) {2 br.readLine();3} catch (IOException e) {4 // do something5}Catch Multiple Exception Types and Rethrowing with Improved Type Checking
Java SE 6
1Class string;2try{3 string = Class.forName("java.lang.String");4 string.getMethod("length").invoke("some error");5} catch(ClassNotFoundException cnfe) {6 System.out.println(cnfe.getMessage());7} catch(IllegalAccessException iae1) {8 System.out.println(iae1.getMessage());9} catch(IllegalArgumentException iae2) {10 System.out.println(iae2.getMessage());11} catch(InvocationTargetException ite) {12 System.out.println(ite.getMessage());13} catch(SecurityException se) {14 System.out.println(se.getMessage());15} catch(NoSuchMethodException nsme) {16System.out.println(nsme.getMessage());17}Java SE 7
1try {2 Class s = Class.forName("java.lang.String");3 s.getMethod("length").invoke("some error");4} catch (ClassNotFoundException | IllegalAccessException5 | IllegalArgumentException | InvocationTargetException6 | SecurityException | NoSuchMethodException e) {7 //Do something here8 System.out.println(e.getMessage());9}
More details about Java SE 7 changes are available on the Java SE 7 Features and Enhancements, especially on the Java Programming Language Enhancements
Can Android Support Java SE 7 Syntax?
According to tools.android.com, you can use most of them with earlier version of Android with targetSDKVersion of 19 and set source compatibility to Java version 1.7, with the exception of Try-with-resources which you must use minSdkVersion of 19 (Android KitKat).
What’s New in Java SE 8 Syntax
Lambda Expressions
1processElements(2 roster,3 p -> p.getGender() == Person.Sex.MALE4 && p.getAge() >= 185 && p.getAge() <= 25,6 p -> p.getEmailAddress(),7 email -> System.out.println(email)8);Improved Type Inference
Type Annotations and Pluggable Type Systems
Repeating Annotations
Method Parameter Reflection
More details of Java SE 8 can be referred to What’s New in JDK 8
Can Android Support Java SE 8 Syntax?
In short (at the time of this blog post), not at the moment, although you can try with unofficial/third party libraries, such as gradle-retrolambda for lambda expressions :)
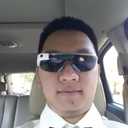
Written by @adrianchia who lives and works in Texas building silly things. You should follow him on Twitter